Qoppa PDF Library jPDFWriter defines a PDFGraphics class which extends Java Graphics2D. Many of the Graphics2D functions are supported (though not all), allowing users to get a graphics object from a PDF page (calling page.createGraphics()) and then drawing onto the page in the same way you would draw to Graphics2D.
Graphics2D has a setPaint() method but in jPDFWriter subclass PDFGraphics, not all paints are supported. You can set the paint to GradientPaint or TexturePaint but LinearGradientPaint and RadialGradientPaint are not supported.
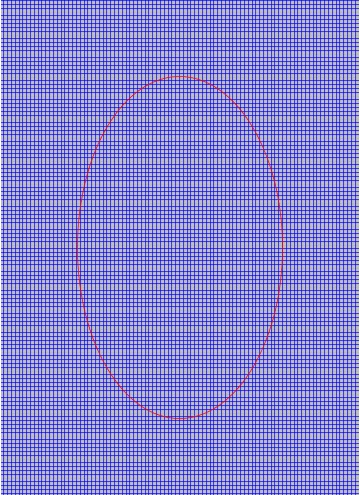
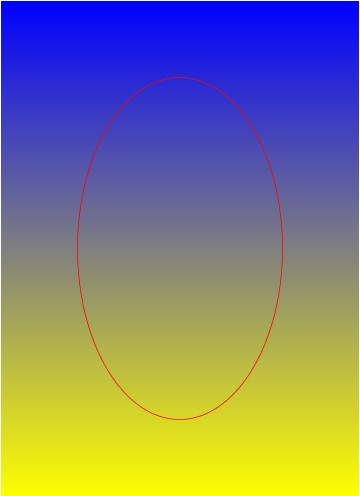
See below for a sample Java program that creates a new PDF documents, add pages to it, sets the paint to either GradientPaint or TexturePaint and draws an oval onto the page.
// create a new PDF PDFDocument pdfDoc = new PDFDocument(); // create a new page format PageFormat pageFormat = new PageFormat(); int x = (int) pageFormat.getImageableX(); int y = (int) pageFormat.getImageableY(); int w = (int) pageFormat.getImageableWidth(); int h = (int) pageFormat.getImageableHeight(); // define paints array Paint[] paints = new Paint[2]; // gradient paint paints[0] = new GradientPaint(x, y, Color.BLUE, x, y + h, Color.YELLOW); // texture paint BufferedImage bi = new BufferedImage(5, 5, BufferedImage.TYPE_INT_RGB); Graphics2D big = bi.createGraphics(); big.setColor(Color.blue); big.fillRect(0, 0, 5, 5); big.setColor(Color.lightGray); big.fillOval(0, 0, 5, 5); Rectangle r = new Rectangle(0, 0, 5, 5); paints[1] = new TexturePaint(bi, r); // linear gradient paint is NOT supported //paints[2] = new LinearGradientPaint(x, y, x, y + h, new float[]{0f, 1f}, new Color[]{Color.BLUE, Color.YELLOW}); // radial gradient paint is NOT supported //paints[3] = new RadialGradientPaint(x + w / 2, y + h / 2, w / 2, new float[]{0f, 1f}, new Color[]{Color.BLUE, Color.YELLOW}); // for each paint for (Paint paint : paints) { // create and add a new page PDFPage page = pdfDoc.createPage(pageFormat); pdfDoc.addPage(page); // get the graphics for this page Graphics2D g = (Graphics2D) page.createGraphics(); // set paint g.setPaint(paint); g.fillRect(x, y, w, h); g.setPaint(Color.RED); // draw an oval g.drawOval(x + 100, y + 100, w - 200, h - 200); } try { pdfDoc.saveDocument("C://test//Gradient.pdf"); } catch(Throwable t) { t.printStackTrace(); } |