This sample will open an existing PDF document, reorganize pages by putting 4 pages of any size onto one single page in letter format (8.5 x 11) and save them as a new PDF document. The pages are organized sequentially in 2 columns and 2 rows, called a sequential imposition layout.
// Load document PDFDocument srcDoc = new PDFDocument("C:/qoppa/test/in.pdf", null); // Create new PDF for output PDFDocument targetDoc = new PDFDocument(); PDFPage targetPage = null; int rows = 2; int cols = 2; for (int i = 0; i < srcDoc.getPageCount(); i++) { // Get source PDF page PDFPage srcPage = srcDoc.getPage(i); int index = i % (rows * cols); if (index == 0) { // Create a new target page targetPage = targetDoc.appendNewPage(8.5 * 72, 11 * 72); } // Calculate the transform to position the page double w = srcPage.getDisplayWidth() * cols; double h = srcPage.getDisplayHeight() * rows; double scale = Math.min(targetPage.getDisplayWidth() / w, targetPage.getDisplayHeight() / h); double tx = srcPage.getDisplayWidth() * scale * (index % cols); double ty = srcPage.getDisplayHeight() * scale * (index / cols); // Overlay PDF content for current source page targetPage.appendPageContent(srcPage, tx, ty, scale, scale, null); } // Save the output document targetDoc.saveDocument("C:/qoppa/test/out.pdf"); |
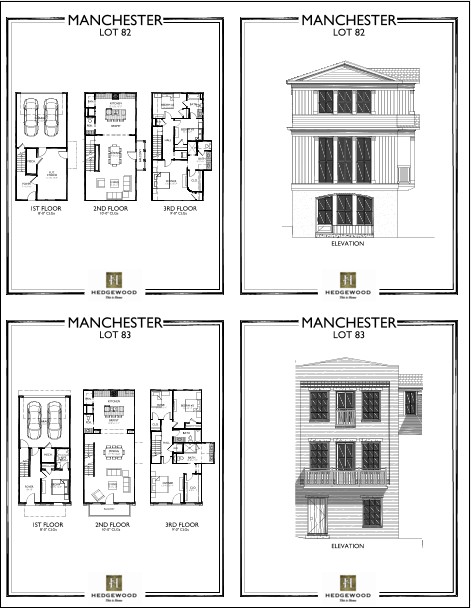
The sample code above can be changed to handle other imposition layouts (such as 2-Up booklet, 4-Up booklet, cut stack, or step and repeat). You can see all the different layouts and binding styles implemented in our end-user desktop PDF editor PDF Studio (look under Document > Imposition).