FormFill is a an activity included in Qoppa’s Android PDF SDK, qPDF Toolkit, that can be used in conjunction with Qoppa’s PDF view for Android, QPDFNotesView, to fill PDF forms fast and efficiently, even on smaller Android phone screens.
It opens as a separate activity that shows all the fields present in the interactive PDF form in a table view. The fields appear in the same order that a user would tab through in the PDF form if it were in a desktop applications.
All the field types are supported including text box, multi-line text box, combo box, checkboxm custom combo box, single list, multi list, etc.
The activity allows to jump to any given page on a the form to fill the fields on this page as well as reset all fields in the form. The activity can also be closed with changes being discarded.
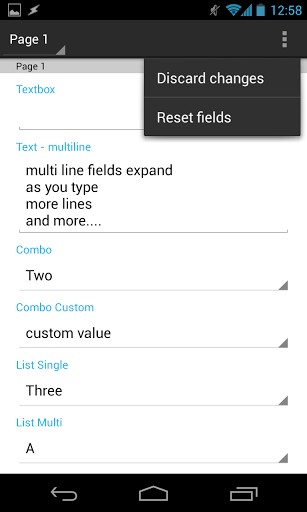
Declare Activity in Manifest
The activity needs to be declared in the application manifest:
<activity
android:name=”com.qoppa.notes.FormFillActivity”
android:configChanges=”keyboardHidden|orientation|keyboard|screenSize”
android:theme=”@android:style/Theme.Light” >
</activity>
Start and Stop the Activity
The FormFill activity needs to be started and stopped from within the main activity. See attached code sample.
package com.qoppa; import com.qoppa.notes.FormFillActivity; import com.qoppa.notes.QPDFNotesView; import android.app.Activity; import android.content.Intent; import android.os.Bundle; import android.view.View; import android.widget.Button; public class MainActivity extends Activity { public static int REQUESTCODE_FORMFILL = 1; public QPDFNotesView notes; protected void onCreate(Bundle bundle) { super.onCreate(bundle); notes = new QPDFNotesView(this); notes.setActivity(this); setContentView(notes); Button formfill = new Button(this); formfill.setText("FormFill"); formfill.setOnClickListener(new View.OnClickListener() { @Override public void onClick(View v) { // Temporarily store the current document so that the fill form activity can access it // Remove it when the activity returns if (notes.getDocument() != null) { FormFillActivity.CURRENT_DOC = notes.getDocument(); Intent intent = new Intent(MainActivity.this, FormFillActivity.class); startActivityForResult(intent, REQUESTCODE_FORMFILL); } } }); notes.getToolbar().addView(formfill); } @Override protected void onActivityResult(int requestCode, int resultCode, Intent data) { super.onActivityResult(requestCode, resultCode, data); if (requestCode == REQUESTCODE_FORMFILL) { // Remove the current doc when we return from the FormFillActivity to prevent multiple documents being in memory simultaneously FormFillActivity.CURRENT_DOC = null; if(resultCode == Activity.RESULT_OK) { // Redraw the content so the fields will reflect the new values notes.redrawContent(); } } } } |