This is a sample java program showing how to sign a PDF document using a certificate loaded from a p12 file. The signature appearance is customized with an image, signer’s name and date. A signature field is added to the PDF document and then signed. This code uses jPDFSecure library but could very easily be adapted to use jPDFProcessby replacing PDFSecure with PDFDocument.
// Load the document PDFSecure pdfDoc = new PDFSecure ("C:\\test\\myfile.pdf", null); // Load the keystore that contains the digital id to use in signing // which can be loaded from a pfx file, a p12 file, etc... FileInputStream pkcs12Stream = new FileInputStream ("C:\\test\\Leila.p12"); KeyStore store = KeyStore.getInstance("PKCS12"); store.load(pkcs12Stream, "mypassword".toCharArray()); pkcs12Stream.close(); // Create signing information using the "Leila" alias SigningInformation signInfo = new SigningInformation (store, "Leila", "mypassword"); // Customize the signature appearance SignatureAppearance signAppear = signInfo.getSignatureAppearance(); // Show an image instead of the signer's name on the left side of the signature field signAppear.setVisibleName(false); signAppear.setImagePosition(SwingConstants.LEFT); signAppear.setImageFile("C:\\test\\image_1.png"); // Only show the signer's name and date on the right side of the signature field signAppear.setVisibleCommonName(false); signAppear.setVisibleOrgUnit(false); signAppear.setVisibleOrgName(false); signAppear.setVisibleLocal(false); signAppear.setVisibleState(false); signAppear.setVisibleCountry(false); signAppear.setVisibleEmail(false); // Create signature field on the first page Rectangle2D signBounds = new Rectangle2D.Double (36, 36, 144, 48); SignatureField signField = pdfDoc.addSignatureField(0, "signature", signBounds); // Apply digital signature pdfDoc.signDocument(signField, signInfo); // Save the document pdfDoc.saveDocument ("C:\\test\\output.pdf"); |
This is a preview of what the electronic signature looks like on the page of the signed PDF:
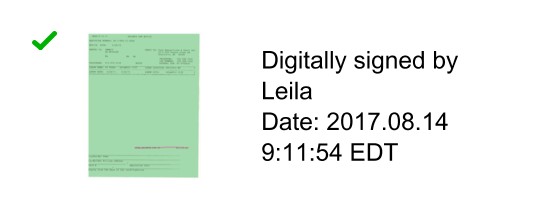