This Java sample code applies a digital signature to a document with a custom appearance. The appearance of signatures can be modified in jPDFSecure to include an image, choose the fields that show or custom text.
/** * Qoppa Software - Sample Source Code */ package jPDFSecureSamples; import java.awt.geom.Rectangle2D; import java.io.FileInputStream; import java.security.KeyStore; import javax.swing.SwingConstants; import com.qoppa.pdf.SignatureAppearance; import com.qoppa.pdf.SigningInformation; import com.qoppa.pdf.form.SignatureField; import com.qoppa.pdfSecure.PDFSecure; public class CustomSignature { public static void main (String [] args) { try { // Load the document PDFSecure pdfDoc = new PDFSecure ("input.pdf", null); // Load the keystore that contains the digital id to use in signing FileInputStream pkcs12Stream = new FileInputStream ("keystore.pfx"); KeyStore store = KeyStore.getInstance("PKCS12"); store.load(pkcs12Stream, "store_pwd".toCharArray()); pkcs12Stream.close(); // Create signing information SigningInformation signInfo = new SigningInformation (store, "key_alias", "key_pwd"); // Set signing properties that will be saved in the signature signInfo.setContactInformation("Phone:404-685-8733"); signInfo.setReason("I approve this document"); signInfo.setLocation("Atlanta, GA"); // Customize the signature appearance SignatureAppearance signAppear = signInfo.getSignatureAppearance(); // LEFT SIDE OF SIGNATURE APPEARANCE // Show an image of handwrittern signature, left justified signAppear.setImagePosition(SwingConstants.LEFT); signAppear.setImageFile("signature.jpg"); // Show visible name which is located on the left side signAppear.setVisibleName(false); // RIGHT SIDE OF SIGNATURE APPEARANCE // Show Digitally Signed By and Date signAppear.setVisibleDigitallySigned(true); signAppear.setVisibleDate(true); // Hide all other fields that usually show on the right side signAppear.setVisibleCommonName(false); signAppear.setVisibleOrgUnit(false); signAppear.setVisibleOrgName(false); signAppear.setVisibleLocal(false); signAppear.setVisibleState(false); signAppear.setVisibleCountry(false); signAppear.setVisibleEmail(false); // Create signature field on the first page Rectangle2D signBounds = new Rectangle2D.Double (36, 36, 144, 48); SignatureField signField = pdfDoc.addSignatureField(0, "signature", signBounds); // Apply digital signature pdfDoc.signDocument(signField, signInfo); // Save the document pdfDoc.saveDocument ("output.pdf"); } catch (Throwable t) { t.printStackTrace(); } } } |
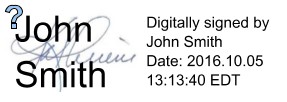