Q: Can I add my own custom annotation tools to Qoppa PDF component’s toolbar?
A: Yes. Qoppa PDF components jPDFNotes and jPDFEditor are fully customizable including the Annotation Toolbar. You can remove any existing tools / buttons from the annotation toolbar and add your own buttons for your own tools. This applies to jPDFNotes and jPDFEditor.
1. First Add Your Own Toolbar Buttons
This sample code shows how to create custom buttoms and add then to the toolbar.
m_NotesBean = new PDFNotesBean(); // button for Free Text Custom Tool JButton jbFreeText = new JButton ("Free Text"); jbFreeText.setForeground(Color.black); jbFreeText.setActionCommand(START_FREETEXT); jbFreeText.addActionListener(this); m_NotesBean.getAnnotToolbar().add(jbFreeText, 0); // button for Circle Custom Tool JButton jbCircle = new JButton ("Circle"); jbCircle.setForeground(Color.red); jbCircle.setActionCommand(START_CIRCLE); jbCircle.addActionListener(this); m_NotesBean.getAnnotToolbar().add (jbCircle, 1); // button for Rubber Stamp Custom Tool JButton jbStamp = new JButton ("Stamp"); jbStamp .setForeground(Color.blue); jbStamp .setActionCommand(START_RUBBERSTAMP); jbStamp .addActionListener(this); m_NotesBean.getAnnotToolbar().add (jbStamp , 2); |
2. Create and Start your own Custom Annotation Tools on Button Click
This is done in the actionPerformed() method, called when the custom buttons are clicked.
public void actionPerformed(ActionEvent e) { MutableDocument doc = m_NotesBean.getMutableDocument(); if(doc != null) { IAnnotationFactory factory = doc.getAnnotationFactory(); // This is a custom FreeText / Text Box Annotation tool if (e.getActionCommand() == START_FREETEXT) { FreeTextTool.setShowPropDialog(false); FreeText correctAnnot = factory.createFreeText("My Text"); m_NotesBean.startEdit (correctAnnot, false, false); } // This is a custom Rubber Stamp Annotation Tool else if (e.getActionCommand() == START_RUBBERSTAMP) { RubberStamp myStamp = factory.createRubberStamp ("My Stamp", Color.blue); myStamp.setRotation(45); m_NotesBean.startEdit (myStamp, false, false); } // This is a custom Circle Annotation Tool else if (e.getActionCommand() == START_CIRCLE) { Circle redCircle = factory.createCircle(null); redCircle.setColor(Color.red); redCircle.setInternalColor(Color.blue); m_NotesBean.startEdit(redCircle, false, false); } } } |
3. See what it looks like
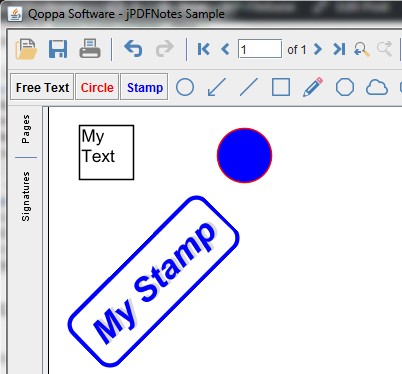
4. Download Full Java Sample
Download full java sample code, showing how to add the component to a frame, add the custom annotation tools and launch the frame.