When running Qoppa Java PDF library jPDFOptimizer to optimize a PDF document and reduce its file size, it is possible to get optimization results of all performed functions as an object called OptimiseResults which allows to programmatically analyze the results and/or output them to a console or to an XML file.
Output Optimizer Results to the Console
// Optimize the document and return optimization results OptimizeResults optimizeResults = pdfOptimize.optimize(options, "C:\\test\\myfile_opt.pdf"); // Write the optimization results to the console if (optimizeResults.getResults() != null) { for (OptimizeResult result : optimizeResults.getResults()) { // recursively write children write(result); } } |
where the write method is the following:
// this method write an OptimizeResult object and its children recursively public static void write(OptimizeResult result) { System.out.println(result.getCount() + " " + result.getDescription()); if (result.getChildren() != null) { for (OptimizeResult curResult : result.getChildren()) write(curResult); } } |
Here is a sample output to the console:
originalBytes 4843764 newBytes 9663955 0 DuplicateImagesMerged 0 DuplicateFontsMerged 0 AltImagesDiscarded 12 LinksDiscarded 0 AnnotationsDiscarded 0 BookmarksDiscarded 1 DocumentInfoDiscarded 0 FileAttachmentsDiscarded 0 FormFieldsDiscarded 1 JSActionsDiscarded 0 ThumbnailsDiscarded 1 XMPMetadataDiscarded 241 ColorImages 237 JPEG (DCTDecode) - JPEG 2000 (0.8) 4 Flate (FlateDecode) - JPEG 2000 (0.8) 1 GrayscaleImages 1 Flate (FlateDecode) - JBIG2 B&W, Black and White 0 BlackAndWhiteImages
Output to an XML file
// Optimize the document and return optimization results OptimizeResults optimizeResults = pdfOptimize.optimize(options, "C:\\test\\myfile_opt.pdf"); // Write the results to an xml file FileOutputStream optOut = new FileOutputStream("c:\\test\\myfile_opt_results.xml"); optimizeResults.writeXml(optOut); optOut.flush(); optOut.close(); |
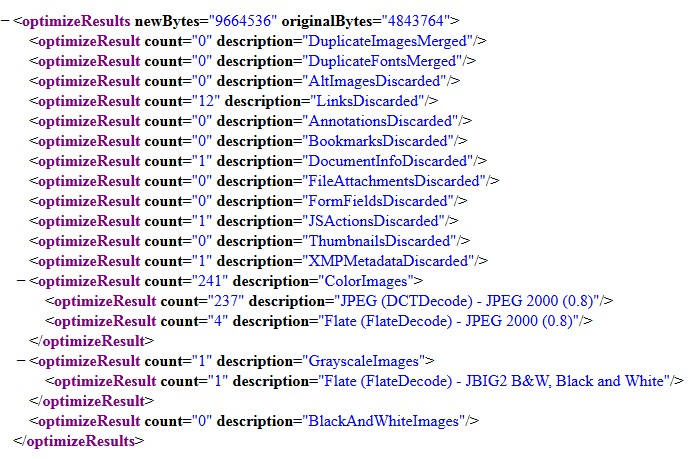